.NET/Unity - Unified Identity: Account Linking & More
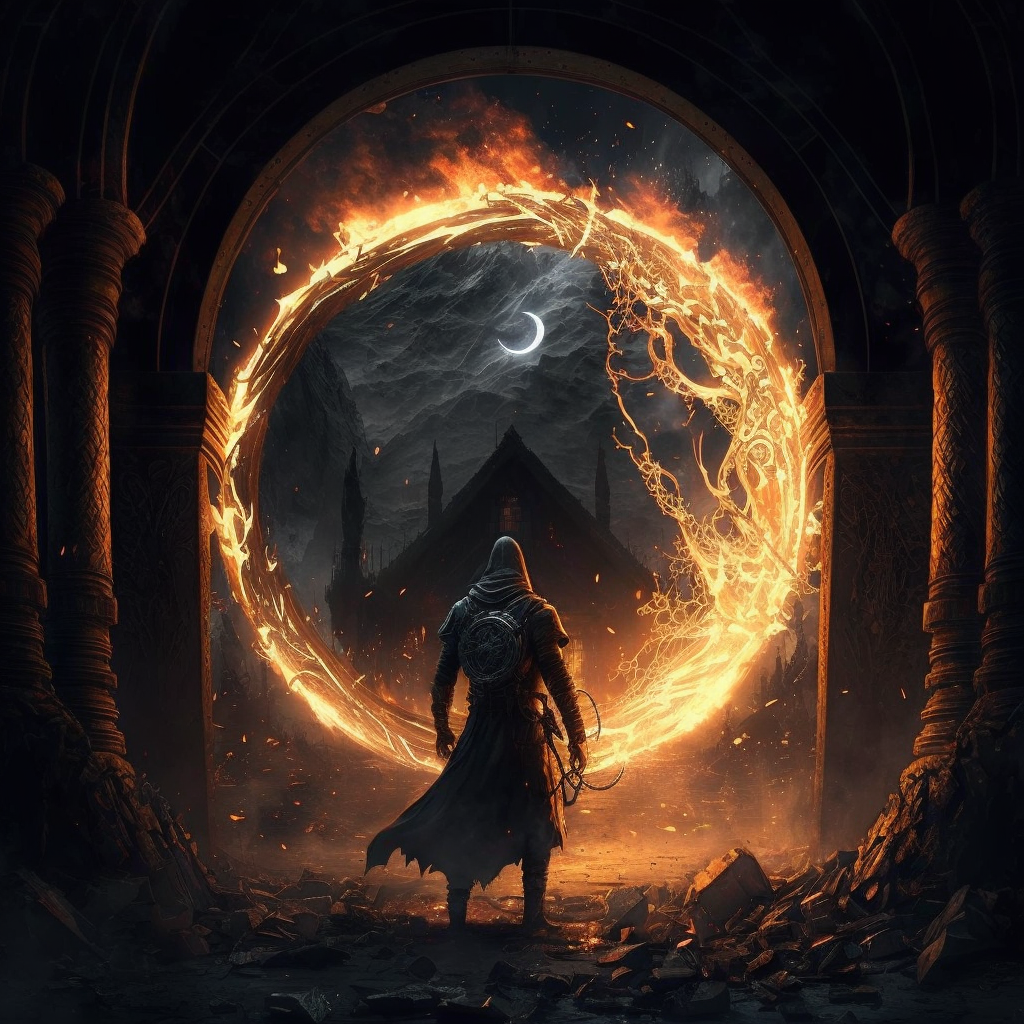
What's Changed
Added SIWE (Sign In With Ethereum) as an additional login provider for In-App Wallets.
Added ability to link accounts, creating a Unified Identity across email, phone, social and other authentication options.
The LinkAccount API requires the parameters you typically use to login with a normal InAppWallet. It will authenticate the new wallet and link it directly to the main wallet. This makes it simple to have multiple identities tied a single evm-compatible account.
Miscellaneous
- Performance and speed improvements for OTP based login methods.
- Added caching for
Utils.FetchThirdwebChainDataAsync
. - Added
Utils.IsEip155Enforced
to check whether a chain enforces EIP 155. - Added smarter transaction gas fee defaults based on whether chain supports 1559.
ThirdwebContract.Read
andThirdwebContract.Write
can now take in full or partial method signatures:- still works.
- now also works.
- now also works.
- now also works.
- We still recommend using our awesome extensions for common standards such as
contract.DropERC20_Claim
to make life easier!
- Added support for ERC20 Paymasters.
Releases
.NET: https://www.nuget.org/packages/Thirdweb/1.4.0
Unity: https://github.com/thirdweb-dev/unity-sdk/releases/tag/v5.0.0-beta.3